AI, zBlog
Natural Language Processing with Python: A Beginner’s Guide with Example Code and Output
atif | Updated: April 26, 2024
Introduction
In today’s data-driven world, the ability to extract meaningful insights from text data is becoming increasingly valuable. Natural Language Processing (NLP) is a branch of artificial intelligence that deals with the interaction between computers and humans using natural languages. NLP techniques enable machines to understand, interpret, and generate human language, making it possible to process and analyze vast amounts of textual data. Python, being a versatile and powerful programming language, has emerged as a popular choice for NLP tasks due to its rich ecosystem of libraries and frameworks. In this comprehensive guide, we’ll explore the fundamentals of Natural Language Processing with Python, covering essential concepts, libraries, and hands-on examples to kickstart your NLP journey.
1. Introduction to Natural Language Processing
Natural Language Processing (NLP) is a multidisciplinary field that combines computer science, linguistics, and machine learning. Its primary goal is to enable computers to understand and process human language in a natural and efficient manner. NLP tasks can be broadly categorized into two main areas:
- Natural Language Understanding (NLU): This involves comprehending and interpreting human language, such as speech recognition, text classification, sentiment analysis, and information extraction.
- Natural Language Generation (NLG): This involves generating human-readable text from structured data, such as text summarization, dialogue systems, and language translation.
NLP has numerous applications across various domains, including customer service (chatbots, virtual assistants), content analysis (sentiment analysis, topic modeling), information retrieval (search engines, question answering), and more.
2. Essential NLP Libraries in Python
Python offers a rich ecosystem of libraries and frameworks for NLP tasks. Here are some of the most popular and widely used libraries:
- NLTK (Natural Language Toolkit): One of the most comprehensive and widely used libraries for NLP in Python, NLTK provides a suite of tools and resources for text processing, including tokenization, stemming, lemmatization, part-of-speech tagging, and more.
- spaCy: A high-performance library for advanced NLP tasks, spaCy offers robust models for tokenization, part-of-speech tagging, named entity recognition, dependency parsing, and more. It is known for its speed and production-ready capabilities.
- Gensim: A robust topic modeling library, Gensim provides efficient implementations of algorithms like Latent Dirichlet Allocation (LDA), Word2Vec, and Doc2Vec for topic modeling, text similarity, and word embeddings.
- TextBlob: A user-friendly library built on top of NLTK and Pattern, TextBlob simplifies common NLP tasks like part-of-speech tagging, noun phrase extraction, sentiment analysis, and more.
- Hugging Face Transformers: A powerful library for state-of-the-art transfer learning models, such as BERT, GPT-2, and XLNet, enabling advanced NLP tasks like text generation, summarization, and question answering.
These libraries provide a solid foundation for building NLP applications in Python, offering a wide range of tools and functionalities to tackle various NLP tasks.
3. Data Preprocessing and Text Cleaning
Before diving into NLP techniques, it’s essential to preprocess and clean the text data to ensure accurate and reliable results. Here are some common preprocessing steps:
import re
import string
def preprocess_text (text):
# Convert to lowercase
text = text.lower()
# Remove URLs
text = re.sub ( r’http\s +’, ‘ ‘ , text)
# Remove HTML tags
text = re.sub ( r'<.*?>’ , ‘ ‘ , text)
# Remove punctuation
text = text.translate( str .maketrans ( ‘ ‘ , ‘ ‘ , string.punctuation ))
# Remove extra whitespace
text = ‘ ‘ .join (text . split() )
return text
In this example, we define a preprocess_text function that performs the following steps:
- Convert the text to lowercase for consistency.
- Remove URLs using regular expressions.
- Remove HTML tags using regular expressions.
- Remove punctuation characters using the string module.
- Remove extra whitespace by joining the words with a single space.
This function can be applied to your text data before proceeding with further NLP tasks.
4. Tokenization and Stemming/Lemmatization
Tokenization is the process of breaking down text into smaller units, such as words or sentences while stemming and lemmatization are techniques for reducing words to their base or root form.
from nltk.tokenize import word_tokenize, sent_tokenize
from nltk.stem import PorterStemmer, WordNetLemmatizer
# Tokenization
text = “This is a sample text for tokenization.”
tokens = word_tokenize(text)
print (tokens)
# Output: [‘This’, ‘is’, ‘a’, ‘sample’, ‘text’, ‘for’, ‘tokenization’, ‘.’]
sentences = sent_tokenize(text)
print(sentences)
# Output: [‘This is a sample text for tokenization.’]
# Stemming
stemmer = PorterStemmer()
stemmed_tokens = [stemmer.stem(token) for token in tokens]
print(stemmed_tokens)
# Output: [‘thi’, ‘is’, ‘a’, ‘sampl’, ‘text’, ‘for’, ‘token’, ‘.’]
# Lemmatization
lemmatizer = WordNetLemmatizer()
lemmatized_tokens = [lemmatizer.lemmatize(token) for token in tokens]
print (lemmatized_tokens)
# Output: [‘This’, ‘is’, ‘a’, ‘sample’, ‘text’, ‘for’, ‘tokenization’, ‘.’]
In this example, we use NLTK’s word_tokenize and sent_tokenize functions to tokenize the text into words and sentences, respectively. We then demonstrate stemming using the PorterStemmer and lemmatization using the WordNetLemmatizer.
5. Part-of-speech tagging and Named Entity Recognition
Part-of-speech (POS) tagging involves assigning a grammatical category (e.g., noun, verb, adjective) to each word in a text, while Named Entity Recognition (NER) aims to identify and classify named entities, such as person names, organizations, and locations.
import spacy
# Load the English language model
nlp = spacy.load(“en_core_web_sm”)
text = “Apple Inc. is an American multinational technology company headquartered in Cupertino, California.”
# Part-of-Speech Tagging
doc = nlp(text)
for token in doc:
print(token.text, token.pos_, token.tag_)
# Named Entity Recognition
print(“Named Entities:”)
for ent in doc.ents:
print(ent.text, ent.label_)
In this example, we use the spaCy library to perform POS tagging and NER. We load the English language model using spacy.load(“en_core_web_sm”) and create a Doc object by passing the text to the nlp object. We then iterate over the tokens in the Doc object and print the token text, part-of-speech tag, and detailed tag.
For Named Entity Recognition, we iterate over the ents (entities) property of the Doc object and print the entity text and label.
6. Sentiment Analysis
Sentiment analysis is the process of determining the sentiment (positive, negative, or neutral) expressed in a piece of text. This can be useful for understanding customer feedback, monitoring brand reputation, and more.
from textblob import TextBlob
text = “This product is amazing! I highly recommend it.”
blob = TextBlob(text)
sentiment = blob.sentiment.polarity
print(f”Sentiment Polarity: {sentiment}“)
# Output: Sentiment Polarity: 0.7
text = “The service was terrible, and the staff was rude.”
blob = TextBlob(text)
sentiment = blob.sentiment.polarity
print(f”Sentiment Polarity: {sentiment}”)
# Output: Sentiment Polarity: -0.6
In this example, we use the TextBlob library to perform sentiment analysis. We create a TextBlob object by passing the text, and then access the sentiment.polarity attribute, which returns a float value between -1 and 1, representing the sentiment of the text (-1 for negative, 0 for neutral, and 1 for positive sentiment). We can use this polarity score to classify the sentiment of the text based on predefined thresholds.
7. Topic Modeling and Document Clustering
Topic modeling is a technique used to discover abstract topics that occur in a collection of documents, while document clustering aims to group similar documents together based on their content.
import gensim
from gensim import corpora
# Sample data
documents = [
“This is a document about machine learning.”,
“Another document on artificial intelligence and deep learning.”,
“A third document discussing natural language processing.”,
“This document covers data mining and big data analytics.”
]
# Create a dictionary from the documents
dictionary = corpora.Dictionary(doc.split() for doc in documents)
# Create a document-term matrix
corpus = [dictionary.doc2bow(doc.split()) for doc in documents]
# Train the LDA model
num_topics = 2
lda_model = gensim.models.LdaMulticore(corpus=corpus, id2word=dictionary, num_topics=num_topics)
# Print the topics and their associated words
print(lda_model.print_topics())
# Compute document similarities
doc1 = documents[0]
doc2 = documents[2]
doc1_bow = dictionary.doc2bow(doc1.split())
doc2_bow = dictionary.doc2bow(doc2.split())
similarity = gensim.matutils.cossim(doc1_bow, doc2_bow)
print(f”Similarity between ‘{doc1}’ and ‘{doc2}’: {similarity}“)
In this example, we use the Gensim library for topic modeling and document clustering. We start by creating a list of sample documents and then build a dictionary and a document-term matrix from these documents.
We then train a Latent Dirichlet Allocation (LDA) model, specifying the number of topics we want to discover. The print_topics method displays the topics and their associated words.
Finally, we demonstrate document similarity by converting two documents into bag-of-words vectors and computing the cosine similarity between them using gensim.matutils.cossim.
These examples scratch the surface of Natural Language Processing with Python, but they provide a solid foundation for understanding and working with text data. As you delve deeper into NLP, you’ll encounter more advanced techniques and applications, such as text generation, machine translation, question answering, and more.
Conclusion
Natural Language Processing with Python is a powerful field that enables machines to understand and interact with human language. By leveraging Python’s rich ecosystem of NLP libraries, you can tackle a wide range of tasks, from text preprocessing and tokenization to sentiment analysis, topic modeling, and document clustering.
This beginner’s guide has provided a comprehensive overview of NLP with Python, covering essential concepts, libraries, and hands-on examples. Whether you’re interested in building chatbots, analyzing customer feedback, or exploring new frontiers in language processing, the knowledge and skills gained from this guide will serve as a strong foundation for your NLP journey.
Trantor is a pioneering company at the forefront of Natural Language Processing (NLP) and Artificial Intelligence (AI) solutions. With a team of highly skilled data scientists, linguists, and software engineers, Trantor is dedicated to developing innovative NLP technologies that empower businesses to unlock the full potential of their textual data.
Leveraging cutting-edge machine learning algorithms and state-of-the-art language models, Trantor offers a comprehensive suite of NLP services, including sentiment analysis, text classification, named entity recognition, information extraction, and more. These solutions are designed to provide invaluable insights and automate complex language-related tasks, enabling organizations to make data-driven decisions and enhance operational efficiency.
Trantor’s commitment to delivering high-quality, tailored solutions sets them apart in the NLP landscape. By combining deep domain expertise with advanced technological capabilities, Trantor ensures that its clients receive customized NLP solutions that address their specific needs and drive meaningful business impact.
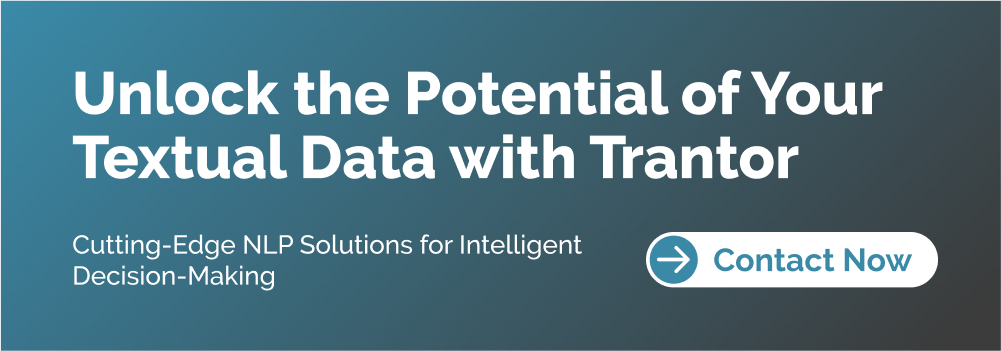